What’s up? I trust you are well.
Allscan Twelve here sharing with you my #100DaysOfCode challenges and we are now at Day 06 Exercise 02. This is just a continuation of our character name “Reeborg” to reach the goal shown with a flag by avoiding obstructions or hurdles, but, this time, with the increase in difficulty.
If you missed the first part, I highly suggest that you read THIS first then come back to this exercise.
So, going back to this second challenge, we know what are the available commands we can use on Reeborg’s basic keyboard. Since there was no turn_right() command, we’ve created a turn_right() function definition and so as the jump() function.
We know from the previous exercise that this code will bring our character Reeborg’s to (13, 1) coordinates. In this next challenge, we never know where is the flag. So, this time, we will be using the “While” loops.
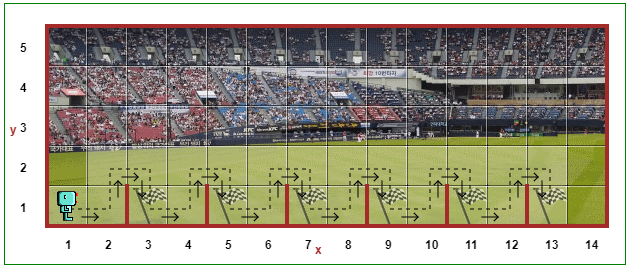
Where do we need to use, “for loop” compared to “while loops”? Good question as I also asked myself that while we were at the beginning of this topic.
“for loops” is used when you have a definite iteration (the number of iterations is known).
For instance, to iterate through a loop with definite range: for hurdle in range(6). With this code, we set and know that this command will run 6 times. It’s gonna stop once it reaches the end of the range.
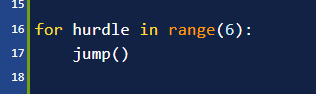
“While loops”, on the other hand, is an indefinite iteration that is used when a loop repeats an unknown number of times and ends when some condition is met. While loop will continue to run for as long as the condition is true. Having said that, “while loops” are a little bit more dangerous than “for loops”. So, if you have a condition that never will become false, for instance, 2 > 1, 2 will always be greater than one and will never return false, so, your while loop becomes something known as an “Infinite Loop”. This is something that, in most cases, you don’t want to happen to your code. If this happens, don’t worry, force quit the program, restart and try to prevent this from happening in the future.
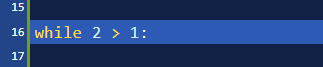
Anyway, let’s go back to Reeborg’s basic keyboard and check what’s on the other tabs. Under “Conditions”, we have these functions. Next, under “Python” we have all of these and we’ve used def(), for in and range() functions as you can see here. Next, we have “Objects”. Maybe we will be using the objects in the next following exercises. And finally under “Specials”, we have these special characters.
Alright. Let’s solve the challenge now using the “while loop”.
Let’s replace all these by while (then, what’s the condition?) at_goal() = False:
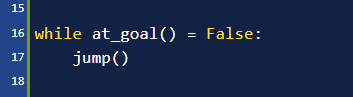
We will tell our character Reeborg’s to jump. Let’s try if this will work.
We have an invalid syntax for our while loop. Let’s review again the syntax for the “while loop”. So, we have the while function then space then, a condition that is something true.
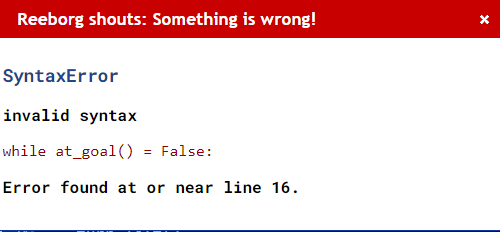
Ok, let’s analyze again the problem.
Let’s try another one to see if this will work.
What about while at_goal() != True
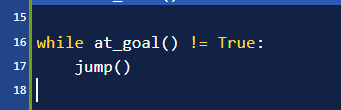
The other way of writing line 16 is (from the instructor’s solution):
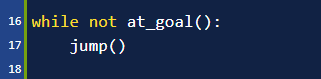
And it worked!
You can watch it in action here:
There you have it. Task completed.
Have a great day. God bless.
Cheers.