The challenge for today is inspired by a game called “FizzBuzz” game where from a given range of numbers, in this case from 1 to 100, we will write a program to satisfy these 3 conditions.
- When the number is divisible by 3 then instead of printing the number it should print “Fizz”.
- When the number is divisible by 5, then instead of printing the number it should print “Buzz”.
- And finally, if the number is divisible by both 3 and 5 e.g. 15 then instead of the number it should print “FizzBuzz”
e.g. it might start off like this:
1 2 Fizz 4 Buzz Fizz 7 8 Fizz Buzz 11 Fizz 13 14 FizzBuzz ....etc
Hint
- Remember your answer should start from 1 and go up to and including 100.
- Each number/text should be printed on a separate line.
My Solution
Let’s start by creating a range of numbers from 1 to 100, inclusive.

Next, if you start by creating an if statement to capture any numbers divisible by 3 or 5, for instance, 15, whoever comes first will be processed first. So if you say:
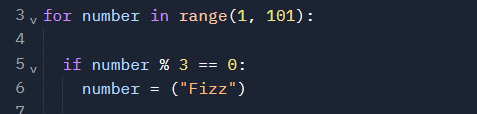
From here the number 15 will be replaced by Fizz which is something that we don’t want for any number that is divisible by 3 and 5.
The best way to capture or processed first any number that is divisible by 3 and 5 is to put them on top before looking at any number that is specifically divisible only to 3 OR 5.
So here is the completed Python code:
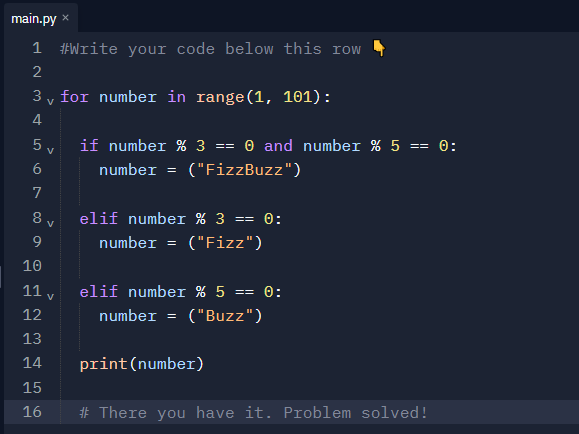
And here is the partial result:
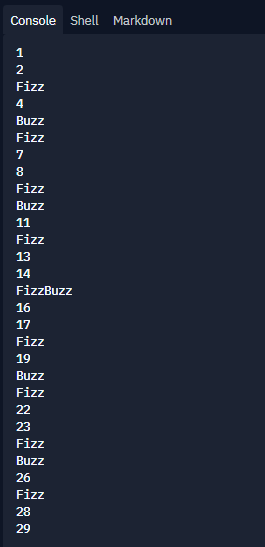
Until then! Have a great day.
Cheers.