The exercise in today’s challenge is to create a Python code to generate a random password from letters, numbers and symbols.
Let’s start by specifying the letters, numbers and symbols that are mostly accepted by any website when creating passwords.
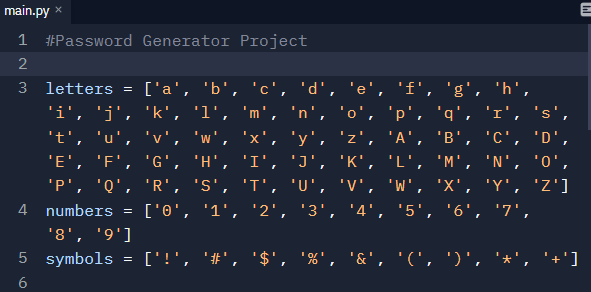
Then some print statements asking the user for their input in terms of how many characters of each kind they want to use for their password.
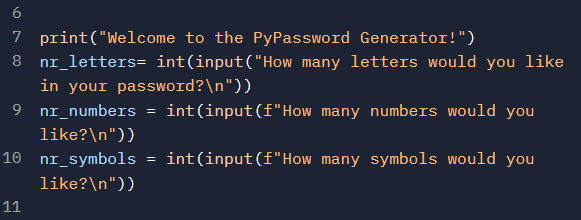
Then we need to import the random module which we need to create some random arrangements by saying:

Then I created 3 “for loops” for my letters, symbols and numbers.
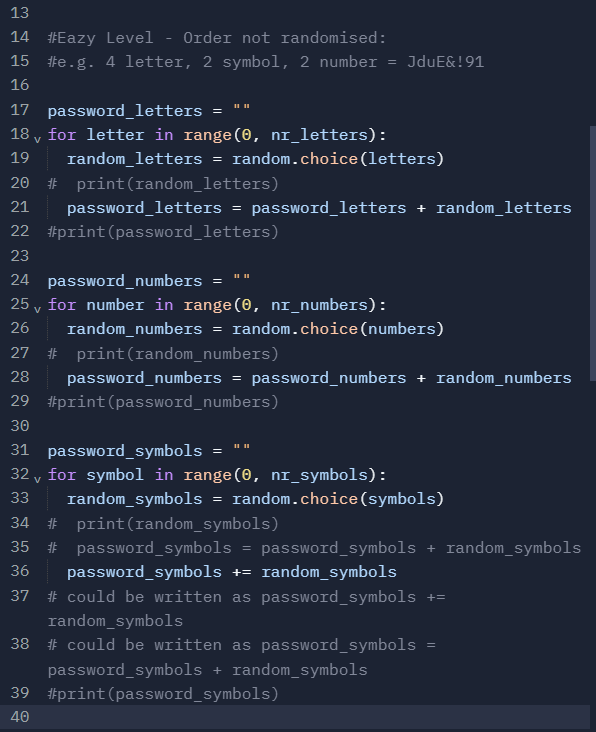
Then I combined them all into a single password in the form of letters+symbols+numbers.

and that completes the easy part of this challenge. The next higher level for this challenge is to randomise these characters to make it difficult to hack by any professional hackers.

I haven’t seen the solution from our instructor but after googling, I found out that I can use the function called random.shuffle(). This function doesn’t work with string. It can’t accept a string argument.
The first thing we need to do is to convert the random_password from line 42 to a list. Let’s do that by saying: new_list = list(random_password)
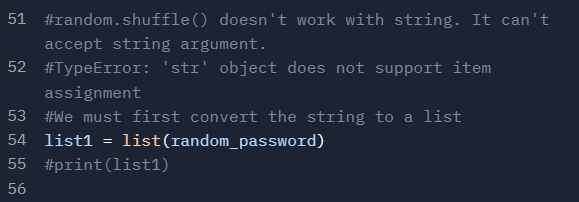
Next, let’s reshuffle it by saying random.shuffle(new_list). I did mine twice here.
Then let’s view the result using the print statement by saying print(new_list).
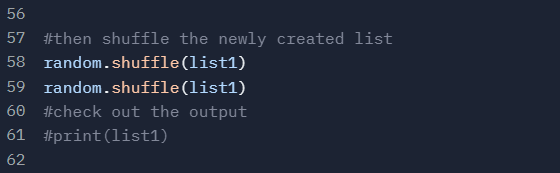
Finally, time to join back those characters in a list using the join() function
Then let’s view the final result using the print statement by saying print(final_password).
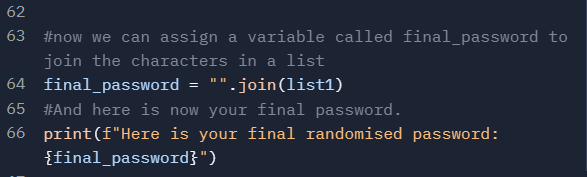
Next, we are ready to run the code.
Here is the sample result of selecting 8 letters, 3 numbers and 5 symbols for the password:
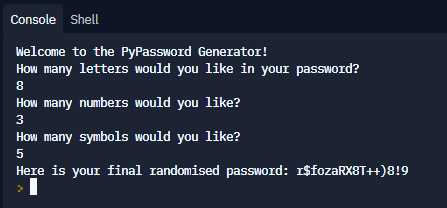
Click the link below to watch this in action:
Until then. Have a great day.
Cheers.